Learning Laravel and angular.js
2015-02-06 - By Robert Elder
Both Laravel and Angular.js are two frameworks that I keep hearing about, so I've decided to take some time to see what they're all about. I created a demo cash flow management app that allows users to sign up for an account, reset passwords, and log in. The code for this is available on GitHub. The cash flow app itself is a single page application that allows users to input simple cash flow items, like client payments or expenses. After these have been input, you'll see the changes on a graph in real time.
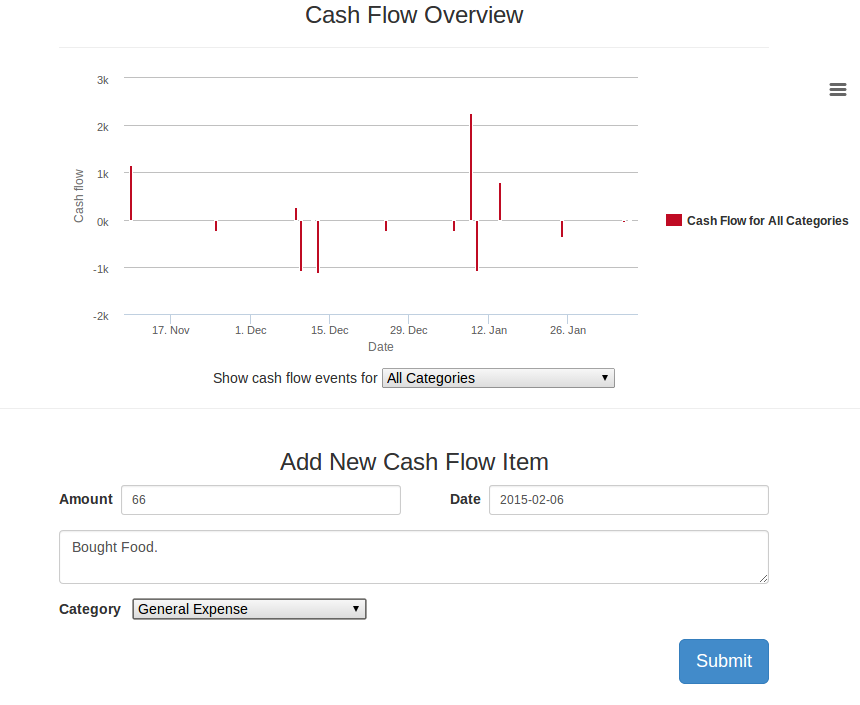
Since Angular.js allows all the different views to be on one page, the application must communicate data for things like editing, or saving through AJAX calls. In any real application, security ends up being something that you need to pay attention to, so I took some time to figure out how to get AJAX calls to properly comply with the Laravel issued CSRF tokens. To do that, I used the following after my "cashflowEventServiceModule" module had been declared:
angular.module("cashflowEventServiceModule").constant("CSRF_TOKEN", '{{ csrf_token() }}');
In the above line, the '{{ csrf_token() }}' is not interpolating the '{{}}'s in Angular.js, but in a Laravel blade.php file, so the rendered result is something like 'kGGRBJGQ6CRHTZ2w8t3HRxWfTEWrbghCM7cvc5ht' when it gets sent to the browser. Then, in order to send the data to the server from Angular.js, you can do
angular.module('cashflowEventServiceModule', [])
.factory('CashflowEvent', function($http, CSRF_TOKEN) {
return {
...
save : function(cashflowEventData) {
var url = ...
cashflowEventData.csrf_json = CSRF_TOKEN;
return $http({
url: url,
data: $.param(cashflowEventData)
});
},
...
}
});
When I was trying to add some changes to one of my migrations in Laravel, I ran into a snag with enum column types. It seems that neither Eloquent or Doctrine ORM has very good support for enum types. One of the errors I got was
[Doctrine\DBAL\DBALException]
Unknown database type enum requested, Doctrine\DBAL\Platforms\MySqlPlatform may not support it.
I found out that this is something that is known about in Doctrine. I didn't find the solution provided on that page very satisfactory, since it seems to require hard-coding enum descriptions for Doctrine itself to use, so I ended up creating the migration to just use raw SQL:
DB::statement("alter table cashflow_events change commenter_id owner_id INT not null;");
One other point of note is that this issue in doctrine, as stated in the link above, claims to only apply if you're renaming the enum, but it also seems to apply if you're renaming any column in a table that contains an enum.
![]() Amazon Cloud Servers For Beginners: Console VS Command-Line
Published 2017-03-20 |
![]() $1.00 CAD |
![]() A Technical Review Of Adding Support For Google's AMP Pages
Published 2016-11-22 |
![]() A Review of the react.js Framework
Published 2015-02-16 |
![]() A Weird Old Tip About EC2 Instances
Published 2015-02-07 |
![]() D3 Visualization Framework
Published 2015-04-21 |
![]() Silently Corrupting an Eclipse Workspace: The Ultimate Prank
Published 2017-03-23 |
![]() XKCD's StackSort Implemented In A Vim Regex
Published 2016-03-17 |
Join My Mailing List Privacy Policy |
Why Bother Subscribing?
|